In the world of embedded systems and IoT devices, power consumption is a critical factor that can make or break the success of a product. The Nordic Power Profiler Kit II (PPK 2) is an indispensable tool for developers looking to optimize the power usage of their devices. While the official nRF Connect Power Profiler provides a user-friendly GUI, there’s a growing need for automation in power monitoring. This is where Python comes into play, offering a way to control PPK 2 programmatically.
Unofficial Python API for PPK 2
An unofficial Python API for PPK 2 has been developed to fill the gap left by the official tool.

This API allows for automated power monitoring and data logging within Python applications, making it possible to integrate power profiling into automated test environments.
Key Features
- Real-time power measurement: The API enables real-time measurement of device power consumption, which is crucial for identifying power spikes and optimizing energy usage.
- Data logging: It supports data logging in user-selectable formats, allowing for long-term power consumption analysis.
- Cross-platform support: The API is designed to work across different platforms, ensuring that developers can use it regardless of their operating system.
Get PCBs for Your Projects Manufactured
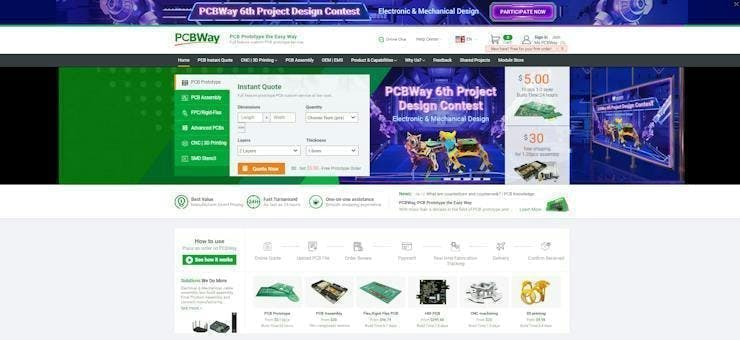
You must check out PCBWAY for ordering PCBs online for cheap!
You get 10 good-quality PCBs manufactured and shipped to your doorstep for cheap. You will also get a discount on shipping on your first order. Upload your Gerber files onto PCBWAY to get them manufactured with good quality and quick turnaround time. PCBWay now could provide a complete product solution, from design to enclosure production. Check out their online Gerber viewer function. With reward points, you can get free stuff from their gift shop. Also, check out this useful blog on PCBWay Plugin for KiCad from here. Using this plugin, you can directly order PCBs in just one click after completing your design in KiCad.
Let's start with UNIHIKER:
The UNIHIKER is a single-board computer that boasts a 2.8-inch touchscreen, providing a tactile and visual interface for your applications. It’s powered by a Quad-Core ARM Cortex-A35 CPU and comes with 512MB RAM and 16GB Flash storage. The board runs on Debian OS, ensuring a familiar and versatile environment for Linux enthusiasts
In this tutorial, we are going to automate the power profiling with the UNIHIKER Single Board Computer.
Install Python API in UNIHIKER:
Connect UNIHER to the PC and open the Mind+ IDE.

Next, connect the UNIHIKER to Mind+ via serial port.

Next, run the following command in the terminal and install the ppk2 library.
pip install ppk2-api

Now you can see the zip file in the UNIHIKER file storage.

Once installed, you can start by importing the necessary classes and initializing the PPK2_API object with the appropriate serial port.
Here’s a basic example to get you started:
import time
from ppk2_api.ppk2_api import PPK2_API
# Initialize the PPK2_API object with the correct serial port
ppk2_test = PPK2_API("/dev/ttyACM3") # Replace with your serial port
# Set up the power profiler
ppk2_test.get_modifiers()
ppk2_test.use_source_meter() # Set source meter mode
ppk2_test.set_source_voltage(3300) # Set source voltage in mV
# Start measuring
ppk2_test.start_measuring()
# Read measured values in a loop
for i in range(0, 1000):
read_data = ppk2_test.get_data()
if read_data != b'':
samples = ppk2_test.get_samples(read_data)
print(f"Average of {len(samples)} samples is: {sum(samples)/len(samples)}uA")
time.sleep(0.001) # Adjust time between sampling as needed
# Stop measuring
ppk2_test.stop_measuring()
This script sets the PPK 2 to source meter mode, starts measuring, and prints out the average current consumption over several samples. It’s a simple yet powerful way to begin automating power profiling for your projects.
Demo
Let's unzip the zip file and open the folder so that you can see the example sketches.

here is the example script.

In this sketch, it will automatically detect the connected PPK2 devices and it will power up the DUT as well as measure the 100 samples.
import time
from ppk2_api.ppk2_api import PPK2_API
from unihiker import GUI # Import the unihiker library
import time # Import the time library
from pinpong.board import Board # Import the Board module from the pinpong.board package
from pinpong.extension.unihiker import * # Import all modules from the pinpong.extension.unihiker package
ppk2s_connected = PPK2_API.list_devices()
if(len(ppk2s_connected) == 1):
ppk2_port = ppk2s_connected[0]
print(f'Found PPK2 at {ppk2_port}')
else:
print(f'Too many connected PPK2\'s: {ppk2s_connected}')
exit()
ppk2_test = PPK2_API(ppk2_port, timeout=1, write_timeout=1, exclusive=True)
ppk2_test.get_modifiers()
ppk2_test.set_source_voltage(3300)
ppk2_test.use_source_meter() # set source meter mode
ppk2_test.toggle_DUT_power("ON") # enable DUT power
ppk2_test.start_measuring() # start measuring
for i in range(0, 100):
read_data = ppk2_test.get_data()
if read_data != b'':
samples, raw_digital = ppk2_test.get_samples(read_data)
print(f"Average is:{sum(samples)/len(samples)}uA")
final=sum(samples)/len(samples)
time.sleep(0.01)
ppk2_test.toggle_DUT_power("OFF") # disable DUT power
ppk2_test.stop_measuring()
Conclusion

The Nordic PPK 2, combined with the power of Python and UNIHIKER, opens up new possibilities for automated power profiling. Whether you’re looking to integrate power profiling into your CI/CD pipeline or simply want to streamline your testing process, the unofficial Python API for PPK 2 is a valuable addition to your toolkit.
I hope this blog post provides a clear overview of how to control the Nordic Power Profiler Kit II with Python and UNIHIKER. If you have any questions or need further assistance, feel free to ask!